How to use dapper with c#
Introduction
Dapper is a simple and efficient micro-ORM (Object Relational Mapping) tool that allows developers to map database objects to C# objects. It is a lightweight and fast library that can be used with any database provider. In this article, we will discuss how to use Dapper with C# to perform CRUD (Create, Read, Update, Delete) operations on a database.
Getting Started with Dapper in C#
Dapper is a lightweight and efficient Object-Relational Mapping (ORM) tool that is widely used in C# applications. It is a simple and easy-to-use tool that allows developers to interact with databases using SQL queries. In this article, we will discuss how to use Dapper with C#.
Firstly, you need to install the Dapper package in your C# project. You can do this by using the NuGet package manager in Visual Studio. Once you have installed the package, you can start using Dapper in your project.
To use Dapper, you need to create a connection to your database. You can do this by using the SqlConnection class in C#. Here is an example of how to create a connection to a SQL Server database using Dapper:
using System.Data.SqlClient;
using Dapper;
SqlConnection connection = new SqlConnection("Server=myServerAddress;Database=myDataBase;User Id=myUsername;Password=myPassword;");
Once you have created a connection, you can start using Dapper to execute SQL queries. Dapper provides a simple and easy-to-use API for executing SQL queries. Here is an example of how to execute a SQL query using Dapper:
string sql = "SELECT * FROM Customers WHERE Country = @Country";
var customers = connection.Query(sql, new { Country = "USA" });
In this example, we are executing a SQL query that selects all customers from the Customers table where the Country column is equal to "USA". We are using the Query method of the Dapper connection object to execute the query. The Query method takes two parameters: the SQL query and a dynamic object that contains the parameters for the query.
Dapper also provides support for executing stored procedures. Here is an example of how to execute a stored procedure using Dapper:
var parameters = new DynamicParameters();
parameters.Add("@CustomerId", 1);
var customer = connection.Query("GetCustomerById", parameters, commandType: CommandType.StoredProcedure).FirstOrDefault();
In this example, we are executing a stored procedure called "GetCustomerById" that takes a parameter called "@CustomerId". We are using the DynamicParameters class to create a dynamic object that contains the parameter for the stored procedure. We are also using the Query method of the Dapper connection object to execute the stored procedure.
Dapper also provides support for mapping query results to objects. Here is an example of how to map query results to objects using Dapper:
public class Customer
{
public int CustomerId { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public string Email { get; set; }
}
string sql = "SELECT * FROM Customers WHERE Country = @Country";
var customers = connection.Query(sql, new { Country = "USA" });
foreach (var customer in customers)
{
Console.WriteLine("CustomerId: {0}, FirstName: {1}, LastName: {2}, Email: {3}", customer.CustomerId, customer.FirstName, customer.LastName, customer.Email);
}
In this example, we have created a Customer class that has properties that match the columns in the Customers table. We are using the Query method of the Dapper connection object to execute the SQL query and map the query results to objects of the Customer class.
In conclusion, Dapper is a simple and easy-to-use ORM tool that is widely used in C# applications. It provides a lightweight and efficient way to interact with databases using SQL queries. By following the examples in this article, you can start using Dapper in your C# projects and take advantage of its many features and benefits.
CRUD Operations with Dapper in C#
Dapper is a micro ORM (Object Relational Mapping) framework that is used to map database objects to C# objects. It is a lightweight and fast framework that is easy to use and can be integrated with any database. Dapper is a popular choice for developers who want to perform CRUD (Create, Read, Update, Delete) operations with C#.
To use Dapper with C#, you need to first install the Dapper NuGet package. You can do this by opening the NuGet Package Manager Console in Visual Studio and typing the following command:
Install-Package Dapper
Once you have installed the Dapper package, you can start using it in your C# code. The first step is to create a connection to your database. You can do this by using the SqlConnection class in C#. Here is an example:
using System.Data.SqlClient;
SqlConnection connection = new SqlConnection("Data Source=your_server_name;Initial Catalog=your_database_name;Integrated Security=True");
Once you have created a connection to your database, you can start using Dapper to perform CRUD operations. The first operation is to retrieve data from the database. You can do this by using the Query method in Dapper. Here is an example:
var customers = connection.Query("SELECT * FROM Customers");
In this example, we are retrieving all the customers from the Customers table in the database. The Query method returns a list of Customer objects that you can use in your C# code.
The next operation is to insert data into the database. You can do this by using the Execute method in Dapper. Here is an example:
var customer = new Customer { Name = "John Doe", Email = "john.doe@email.com" };
connection.Execute("INSERT INTO Customers (Name, Email) VALUES (@Name, @Email)", customer);
In this example, we are inserting a new customer into the Customers table in the database. The Execute method takes a SQL query and a parameter object as input. The parameter object contains the values that you want to insert into the database.
The next operation is to update data in the database. You can do this by using the Execute method in Dapper. Here is an example:
var customer = new Customer { Id = 1, Name = "John Doe", Email = "john.doe@email.com" };
connection.Execute("UPDATE Customers SET Name = @Name, Email = @Email WHERE Id = @Id", customer);
In this example, we are updating the name and email of a customer with the Id of 1 in the Customers table in the database. The Execute method takes a SQL query and a parameter object as input. The parameter object contains the values that you want to update in the database.
The final operation is to delete data from the database. You can do this by using the Execute method in Dapper. Here is an example:
connection.Execute("DELETE FROM Customers WHERE Id = @Id", new { Id = 1 });
In this example, we are deleting a customer with the Id of 1 from the Customers table in the database. The Execute method takes a SQL query and a parameter object as input. The parameter object contains the values that you want to delete from the database.
In conclusion, Dapper is a powerful and easy-to-use framework for performing CRUD operations with C#. It is a lightweight and fast framework that can be integrated with any database. By following the examples in this article, you can start using Dapper in your C# code and perform CRUD operations with ease.
Advanced Dapper Techniques for C# Developers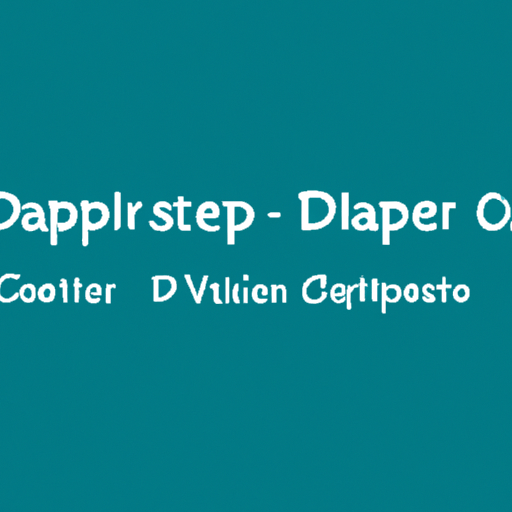
Dapper is a popular micro-ORM (Object-Relational Mapping) tool that is widely used by C# developers. It is a lightweight and fast tool that allows developers to map database tables to C# objects. Dapper is easy to use and provides a lot of features that make it a great choice for developers who want to work with databases in C#.
In this article, we will discuss some advanced techniques for using Dapper with C#. These techniques will help you to get the most out of Dapper and make your code more efficient and effective.
Firstly, let's talk about how to use Dapper with stored procedures. Stored procedures are precompiled SQL statements that are stored in the database. They are used to perform complex database operations and are often used in enterprise-level applications. Dapper provides a simple way to execute stored procedures in C#. To use a stored procedure with Dapper, you need to create a command object and set its CommandType property to CommandType.StoredProcedure. You can then pass the name of the stored procedure and any parameters to the command object and execute it using the Execute method.
Another advanced technique for using Dapper is to use dynamic parameters. Dynamic parameters allow you to pass parameters to a SQL statement without having to specify their data type. This can be useful when you are working with complex SQL statements that have a lot of parameters. To use dynamic parameters with Dapper, you need to create a DynamicParameters object and add your parameters to it using the Add method. You can then pass the DynamicParameters object to the Query or Execute method and Dapper will automatically map the parameters to the SQL statement.
Dapper also provides support for asynchronous database operations. Asynchronous operations allow you to perform database operations without blocking the main thread of your application. This can be useful when you are working with large amounts of data or when you need to perform multiple database operations at the same time. To use asynchronous operations with Dapper, you need to use the async and await keywords in your code. You can then use the QueryAsync or ExecuteAsync method to perform asynchronous database operations.
Another advanced technique for using Dapper is to use the multi-mapping feature. Multi-mapping allows you to map multiple database tables to a single C# object. This can be useful when you are working with complex database schemas that have multiple tables. To use multi-mapping with Dapper, you need to create a custom mapping function that maps the database tables to the C# object. You can then pass the mapping function to the Query method and Dapper will automatically map the database tables to the C# object.
Finally, let's talk about how to use Dapper with transactions. Transactions are used to ensure that a group of database operations are performed as a single unit of work. This can be useful when you need to perform multiple database operations that depend on each other. To use transactions with Dapper, you need to create a transaction object and pass it to the Query or Execute method. You can then use the Commit or Rollback method to commit or rollback the transaction.
In conclusion, Dapper is a powerful tool that can help you to work with databases in C#. By using these advanced techniques, you can get the most out of Dapper and make your code more efficient and effective. Whether you are working with stored procedures, dynamic parameters, asynchronous operations, multi-mapping, or transactions, Dapper provides a simple and easy-to-use interface that makes it a great choice for C# developers.
Using Dapper with ASP.NET Core
Dapper is a lightweight and efficient Object-Relational Mapping (ORM) tool that is widely used in the .NET community. It is a simple and easy-to-use tool that allows developers to map database tables to C# objects. Dapper is known for its speed and performance, making it a popular choice for developers who want to build fast and efficient applications.
If you are working with ASP.NET Core, you can easily integrate Dapper into your project. In this article, we will discuss how to use Dapper with ASP.NET Core.
First, you need to install the Dapper package. You can do this by using the NuGet package manager in Visual Studio or by running the following command in the Package Manager Console:
Install-Package Dapper
Once you have installed the Dapper package, you can start using it in your ASP.NET Core project. The first step is to create a connection string to your database. You can do this in the appsettings.json file in your project. Here is an example of a connection string:
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)mssqllocaldb;Database=MyDatabase;Trusted_Connection=True;MultipleActiveResultSets=true"
}
Next, you need to create a class that represents the table in your database. For example, if you have a table called "Customers" in your database, you can create a class called "Customer" that has properties that match the columns in the table. Here is an example of a Customer class:
public class Customer
{
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
Once you have created your class, you can use Dapper to query the database and map the results to your class. Here is an example of how to retrieve all customers from the database:
using (var connection = new SqlConnection(Configuration.GetConnectionString("DefaultConnection")))
{
connection.Open();
var customers = connection.Query("SELECT * FROM Customers");
return View(customers);
}
In this example, we are using the SqlConnection class to create a connection to the database. We then use the Query method to execute a SQL query and map the results to a list of Customer objects.
You can also use Dapper to insert, update, and delete records in the database. Here is an example of how to insert a new customer into the database:
using (var connection = new SqlConnection(Configuration.GetConnectionString("DefaultConnection")))
{
connection.Open();
var customer = new Customer { Name = "John Doe", Email = "johndoe@example.com" };
var sql = "INSERT INTO Customers (Name, Email) VALUES (@Name, @Email)";
var affectedRows = connection.Execute(sql, customer);
return RedirectToAction("Index");
}
In this example, we are using the Execute method to execute an SQL query that inserts a new customer into the database. We pass in a Customer object as a parameter, and Dapper automatically maps the properties of the object to the corresponding columns in the table.
In conclusion, Dapper is a powerful and efficient ORM tool that can be easily integrated into your ASP.NET Core project. By following the steps outlined in this article, you can start using Dapper to query, insert, update, and delete records in your database. With its speed and performance, Dapper is a great choice for developers who want to build fast and efficient applications.
Performance Optimization with Dapper in C#
Dapper is a micro ORM (Object-Relational Mapping) framework that is designed to provide fast data access while keeping the code simple and easy to maintain. It is a lightweight and efficient tool that can be used with C# to optimize performance and improve the overall user experience. In this article, we will discuss how to use Dapper with C# to achieve performance optimization.
Firstly, it is important to understand the basics of Dapper. Dapper is a simple and easy-to-use ORM that provides a set of extension methods to execute SQL queries and map the results to C# objects. It is built on top of ADO.NET and provides a lightweight and efficient way to access data from a database. Dapper is designed to be fast and efficient, and it achieves this by using dynamic SQL generation and object mapping.
To use Dapper with C#, you need to install the Dapper NuGet package. You can do this by opening the NuGet Package Manager in Visual Studio and searching for Dapper. Once you have installed the package, you can start using Dapper in your C# code.
One of the key features of Dapper is its ability to map database columns to C# properties. This means that you can write SQL queries that return data from a database and map the results to C# objects. To do this, you need to define a class that represents the data you want to retrieve from the database. The class should have properties that match the columns in the database table.
For example, if you have a table called Customers with columns called Id, Name, and Email, you can define a class called Customer with properties called Id, Name, and Email. You can then write a SQL query that retrieves data from the Customers table and maps the results to a list of Customer objects.
To execute the SQL query and map the results to C# objects, you can use the Query method provided by Dapper. The Query method takes two parameters: the SQL query and an object that represents the parameters for the query. The parameters object can be a simple anonymous type or a more complex object that represents the parameters for the query.
For example, to retrieve all customers from the Customers table, you can write the following code:
using (var connection = new SqlConnection(connectionString))
{
var customers = connection.Query("SELECT Id, Name, Email FROM Customers").ToList();
}
This code creates a new SqlConnection object and opens a connection to the database using the connection string. It then executes a SQL query that retrieves data from the Customers table and maps the results to a list of Customer objects using the Query method provided by Dapper.
Another key feature of Dapper is its ability to execute stored procedures. Stored procedures are precompiled SQL statements that are stored in a database and can be executed by calling a stored procedure name. Stored procedures can be used to improve performance by reducing the amount of data that needs to be transferred between the database and the application.
To execute a stored procedure using Dapper, you can use the Query method and specify the stored procedure name as the SQL query. You can also specify the CommandType as StoredProcedure to indicate that you are executing a stored procedure.
For example, to execute a stored procedure called GetCustomers, you can write the following code:
using (var connection = new SqlConnection(connectionString))
{
var customers = connection.Query("GetCustomers", commandType: CommandType.StoredProcedure).ToList();
}
This code creates a new SqlConnection object and opens a connection to the database using the connection string. It then executes a stored procedure called GetCustomers and maps the results to a list of Customer objects using the Query method provided by Dapper.
In conclusion, Dapper is a powerful and efficient tool that can be used with C# to optimize performance and improve the overall user experience. By using Dapper, you can write simple and easy-to-maintain code that provides fast data access and reduces the amount of data that needs to be transferred between the database and the application. With its ability to map database columns to C# properties and execute stored procedures, Dapper is a valuable tool for any C# developer looking to improve performance.
Conclusion
To use Dapper with C#, you need to first install the Dapper package using NuGet. Then, you can create a connection to your database and use Dapper's extension methods to execute SQL queries and map the results to C# objects. Dapper is a lightweight and efficient ORM tool that can help simplify database interactions in C# applications.
Leave a Reply